Hey all, I have a simple map in my game.
The map icons are turned on by a simple True statement, and turned off and go grey with a simple False statement. When you have visited them all another simple visit statement sends you home automatically. Now that's all fine and dandy.
In this chapter however, one day you go visit the places, and some random events happen, the following day when the map comes up 2,3,4 or all 5 places may or may not show, depending on the random event.
So I don't know when the player has visited everything as I have no idea what was on their map. The only thing I do know, is whatever came up on their map was triggered by a True statement, or it would not have showed up.
So what I want to know -
Player 1 has three places to visit, so he has triggered 3 location = True statements
Player 2 has four places to visit, so he has triggered 4 location = True statements
Player 3 has five places to visit, so he has triggered 5 location = True statements
Is there way to tell renpy when all location = True statements have been triggered to do something, regardless of how many there is.
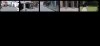
The map icons are turned on by a simple True statement, and turned off and go grey with a simple False statement. When you have visited them all another simple visit statement sends you home automatically. Now that's all fine and dandy.
In this chapter however, one day you go visit the places, and some random events happen, the following day when the map comes up 2,3,4 or all 5 places may or may not show, depending on the random event.
So I don't know when the player has visited everything as I have no idea what was on their map. The only thing I do know, is whatever came up on their map was triggered by a True statement, or it would not have showed up.
So what I want to know -
Player 1 has three places to visit, so he has triggered 3 location = True statements
Player 2 has four places to visit, so he has triggered 4 location = True statements
Player 3 has five places to visit, so he has triggered 5 location = True statements
Is there way to tell renpy when all location = True statements have been triggered to do something, regardless of how many there is.