Ok, I'm trying to do something, and I'm still working out a reasonable way to code it. (Note: I do have a couple alternative ideas if this one doesn't pan out or isn't easily feesible, but I would like to get this one to work). First, some visual aids.
Ok, so behold my amazing MS paint skills.
Looking at this image, let's ignore 2 things - the grid, and the yellow blob. So it's just a white screen and 2 red blobs - that represents the render being used as part of the background. The grid wouldn't be present in the game, that's only there to make this example easier to understand.
The yellow blob represents an imagebutton. In theory I want do do the following:
#1: break the screen up into a 3x3 grid (so 9 zones). This would be done on the back end, not visually - the grid wouldn't actually be displayed.
#2: Create an imagebutton that moves around the screen. Simple enough with a transform. Preferrably semi-randomly, but not necessary.
#3
optional) determine (So far I think I'd have to determine it beforehand and hardcode this bit in) the positions of the "red blobs" (Again, these are not objects in the programming sense, they're just parts of the background image). The goal is that when the imagebutton moves around, it avoids areas currently inhabited by the red blobs. There would be multiple images, with the red blobs in different positions in each image, so my only thought now would be to determine beforehand their exact positions on screen, and hardcode that into the transform somehow, so that the movement of the imagebutton avoids those exact spots.
#4: The above 3 steps I at least have some idea of how to accomplish, but so far I haven't come up with this step yet. Still working on it. Detect what "zone" the imagebutton is in, at time of click. Preform an action based on the position of the button at the time. The action itself would also be variable based on the position of the red dots, but that part is much easier, it's the positional detection of the button at time of click I haven't figured out yet.
So that's the basics of what I want to do. Have an imagebutton move around the screen semi-randomly, have it avoid specific areas that would most likely be pre-defined (But variable depending on what image is currently loaded), and have variable actions preformed based on the location of the button when it's clicked.
I'll be working on this myself on and off for a bit, but if anyone has any ideas I'd appreciate it.
Edit: so the first thing I came across when looking around was this:
While not exactly what I need, it's close enough that I should be able to use that as a basis to get the exact mouse position at the time the imagebutton is clicked.
If that worked, then I'd just need the imagebutton action to call a function and pass the x/y coords to it - that function would need to be capable of processing the coords and preforming the variable action depending on their positioning. Ideally I'd also want to "Mark off" zones somehow within that function, that are inhabited by the red dots.
So using the above image for an example, calling the top left zone 1, top right zone 3, bottom right zone 9, etc...
Zone 1,4,6 and 9 are currently inhabited, zone 2,3,5,6,8 are empty. If the button was clicked while in zones 2,3,5,6,8, preform 1 action. If the button was clicked while in zone 1,4,6 or 9, preform a different action. This can't just be statically defined, since in theory the position of the red dots would change every time the image changed.
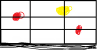
Ok, so behold my amazing MS paint skills.
Looking at this image, let's ignore 2 things - the grid, and the yellow blob. So it's just a white screen and 2 red blobs - that represents the render being used as part of the background. The grid wouldn't be present in the game, that's only there to make this example easier to understand.
The yellow blob represents an imagebutton. In theory I want do do the following:
#1: break the screen up into a 3x3 grid (so 9 zones). This would be done on the back end, not visually - the grid wouldn't actually be displayed.
#2: Create an imagebutton that moves around the screen. Simple enough with a transform. Preferrably semi-randomly, but not necessary.
#3
#4: The above 3 steps I at least have some idea of how to accomplish, but so far I haven't come up with this step yet. Still working on it. Detect what "zone" the imagebutton is in, at time of click. Preform an action based on the position of the button at the time. The action itself would also be variable based on the position of the red dots, but that part is much easier, it's the positional detection of the button at time of click I haven't figured out yet.
So that's the basics of what I want to do. Have an imagebutton move around the screen semi-randomly, have it avoid specific areas that would most likely be pre-defined (But variable depending on what image is currently loaded), and have variable actions preformed based on the location of the button when it's clicked.
I'll be working on this myself on and off for a bit, but if anyone has any ideas I'd appreciate it.
Edit: so the first thing I came across when looking around was this:
Code:
default mouse_xy = (0, 0)
init python:
def get_mouse():
global mouse_xy
mouse_xy = renpy.get_mouse_pos()
screen mouse_test()
on "show" action Function(get_mouse)
text "[mouse_xy[0]], [mouse_xy[1]]"
timer 0.01 repeat True action Function(get_mouse)
key "mousedown_1" action Show("spark", x=mouse_xy[0], y=mouse_xy[1])
While not exactly what I need, it's close enough that I should be able to use that as a basis to get the exact mouse position at the time the imagebutton is clicked.
If that worked, then I'd just need the imagebutton action to call a function and pass the x/y coords to it - that function would need to be capable of processing the coords and preforming the variable action depending on their positioning. Ideally I'd also want to "Mark off" zones somehow within that function, that are inhabited by the red dots.
So using the above image for an example, calling the top left zone 1, top right zone 3, bottom right zone 9, etc...
Zone 1,4,6 and 9 are currently inhabited, zone 2,3,5,6,8 are empty. If the button was clicked while in zones 2,3,5,6,8, preform 1 action. If the button was clicked while in zone 1,4,6 or 9, preform a different action. This can't just be statically defined, since in theory the position of the red dots would change every time the image changed.
Last edited: