- Sep 29, 2020
- 58
- 1,133
Hey,
I have the most weird bug I've encountered so far.
I'm building a scene gallery with support for multi-part scenes. Multi-part scenes basically mean that you can obtain only certain parts of a scene and miss others. You might not have had enough points for... stuff.
The checkpoints are stored in persistent variables, along with the
The checkpoints are displayed under the thumbnail of the scene in the gallery. This can either be a checkmark or an X, for simplicity's sake.
Since I'm using custom classes to store the scene item's information, I had to declare the class in an
The problem is that when the gallery screen is parsing through the scene item's
Here is the code, for clarity:
This is the Gallery implementation, the key point is how it parses through the
This is the SceneItem implementation:
GalleryItems are created and appended as such (check the last item of the constructor, the list of variables):
The weird part is that when running this code, the behaviour is SUPER awkward. I dunno why. Since the screen 'functions' run multiple times, the print statements also do. And the results are inconsistent. No clue why. Here is the latest log:
As you can see, during the second pass, the iterations run more then they should and the code somehow enters the "unlocked" statement, even when the boolean value is false (which it shouldn't be). You can see it at the end of the sentence. "Adding UNLOCKED button with scene element Sicily, boolean value is False". This should never happen.
I'd appreciate any help which would bring me closer to figuring out this issue.
I have the most weird bug I've encountered so far.
I'm building a scene gallery with support for multi-part scenes. Multi-part scenes basically mean that you can obtain only certain parts of a scene and miss others. You might not have had enough points for... stuff.
The checkpoints are stored in persistent variables, along with the
SceneItem
classes as well. This class holds every data related to a scene.The checkpoints are displayed under the thumbnail of the scene in the gallery. This can either be a checkmark or an X, for simplicity's sake.
Since I'm using custom classes to store the scene item's information, I had to declare the class in an
init python early
block (to be able to persist the class). I imagine this is causing my problem right now.The problem is that when the gallery screen is parsing through the scene item's
multi_part_unlocks
list (which is a list of persisted boolean variables), it seemingly ignores the persistent variable's value and defaults to false.Here is the code, for clarity:
This is the Gallery implementation, the key point is how it parses through the
multi_part_unlocks
Python:
init 501 screen scenegallery():
tag menu
python:
import math
GALLERY_SIZE = len(persistent.scene_list)
GALLERY_COLUMNS = 3
GALLERY_ROWS = int(math.ceil((float(GALLERY_SIZE) / GALLERY_COLUMNS)))
EMPTY_COUNT = GALLERY_ROWS * GALLERY_COLUMNS - GALLERY_SIZE
use game_menu(_("Scenene Gallery"), scroll="viewport"):
style_prefix "underconst"
$ print ("-----------------------")
$ print("Adding scene gallery")
$ print ("-----------------------")
vbox:
label "Replay your favourite moments"
null height 50
grid GALLERY_COLUMNS GALLERY_ROWS:
spacing 30
for scene_element in persistent.scene_list:
if scene_element.is_locked:
# Add locked image with no actions.
vbox:
text _(scene_element.item_name) xalign 0.5
imagebutton:
idle scene_element.locked_image
else:
# Add unlocked hover/idle image and action
vbox:
text _(scene_element.item_name) xalign 0.5
imagebutton:
idle scene_element.unlocked_idle_image
hover scene_element.unlocked_hover_image
action Replay(scene_element.label_name, locked = False)
if len(scene_element.multi_part_unlocks) > 0:
$ print("The following scene element IS multi-part: ", scene_element.item_name)
hbox:
for persistent_variable in scene_element.multi_part_unlocks:
if persistent_variable == True:
$ print("Adding UNLOCKED button with scene element ", scene_element.item_name, "boolean value is", (persistent_variable))
add "multi_part_unlocked"
else:
$ print("Adding LOCKED button with scene element ", scene_element.item_name,"boolean value is", (persistent_variable))
add "multi_part_locked"
else:
$ print("The following scene element IS NOT multi-part: ", scene_element.item_name)
for i in range(EMPTY_COUNT):
null
Python:
# The SceneItem class is the basic model of a single element in the scene gallery
# item_id - Unique ID
# item_name - Item name shown in the Scene Gallery
# is_unlocked_by_default - Determines whether the item should be unlocked by default or not.
# unlocked_idle_image - Unlocked image resource, idle state
# unlocked_hover_image - Unlocked image resource, hover state
# locked_image - Locked image resource, has no states
# label_name - The label's name referred to, for replay.
# multi_part_unlocks - A list persistent boolean variables that control how much of the multi-part scene has been unlocked
init python early:
class SceneItem:
def __init__(self, item_id, item_name, is_unlocked_by_default, unlocked_idle_image, unlocked_hover_image, locked_image, label_name, multi_part_unlocks):
self.item_id = item_id
self.item_name = item_name
self.unlocked_idle_image = unlocked_idle_image
self.unlocked_hover_image = unlocked_hover_image
self.locked_image = locked_image
self.label_name = label_name
self.is_locked = not is_unlocked_by_default
self.multi_part_unlocks = multi_part_unlocks
def __eq__(self, other):
return self.item_id == other.item_id
def unlock(self):
self.is_locked = False
Python:
if persistent.scene_list is None:
persistent.scene_list = []
persistent.scene_list.append(SceneItem(1, "Sicily", True, "gallery_item_sicily_idle", "gallery_item_sicily_hover", "gallery_item_locked", "IntSicilyLewd", [persistent.fiona_other_stuff, persistent.fiona_another_stuff]))
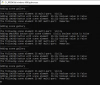
As you can see, during the second pass, the iterations run more then they should and the code somehow enters the "unlocked" statement, even when the boolean value is false (which it shouldn't be). You can see it at the end of the sentence. "Adding UNLOCKED button with scene element Sicily, boolean value is False". This should never happen.
I'd appreciate any help which would bring me closer to figuring out this issue.
Last edited: