Heroine Hunter X
Newbie
- Jan 22, 2020
- 59
- 157
I've been working on my game adding new features as I go. As the title suggests, the game is developed in Twine using Tweego and SugarCube. I've reached a stumbling block pertaining to location maps (minimap?). Spent a few days researching various solutions and landed on HTML5 canvases. Not in love with it, doesn't want to play with Tweego whatsoever, but I'm coming up empty. I've seen others implement this feature so I'm hoping for some insight here. The best way I can describe what I'm after is an old school Zelda dungeon map with black boxes representing rooms. Here is my canvas code that achieved the basics of the appearance.
The result looks like this:
This is obviously a mockup and has no further functionality. I can script how to move the player around, but it would be nice to have something visual to represent it. No clue how others are achieving this in SugarCube. Any thoughts and ideas would be greatly appreciated.
HTML:
<!DOCTYPE html>
<html>
<head>
<title>GAME</title>
<style>
body,
html {
background-color: black;
width: 100%;
height: 100%;
padding: 0;
margin: 0;
}
#canvas {
border: 1px solid black;
background-color: rgb(49, 49, 49);
width: 512px;
height: 288px;
}
#container {
width: 512px;
height: 288px;
margin: auto;
}
</style>
</head>
<body>
<div id="container">
<canvas id="canvas" height="288px" width="512px"></canvas>
</div>
<script>
var canvas = document.getElementById("canvas");
var context = canvas.getContext("2d");
var mapArray = [
[-1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1],
[-1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1],
[-1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1],
[-1, -1, -1, -1, -1, -1, 0, -1, -1, 0, 0, -1, -1, -1, -1, -1],
[-1, -1, -1, -1, -1, 0, 0, -1, -1, 0, -1, -1, -1, -1, -1, -1],
[-1, -1, -1, -1, -1, -1, 0, 0, 0, 0, 0, 0, -1, -1, -1, -1],
[-1, -1, -1, -1, -1, -1, -1, 0, -1, -1, 0, -1, -1, -1, -1, -1],
[-1, -1, -1, -1, -1, -1, -1, 0, -1, 0, 0, -1, -1, -1, -1, -1],
[-1, -1, -1, -1, -1, -1, -1, 1, -1, -1, -1, -1, -1, -1, -1, -1],
];
var posX = 0;
var posY = 0;
var room = new Image();
room.src = "room.png";
room.onload = mapDraw;
var redBox = new Image();
redBox.src = "redBox.png";
redBox.onload = mapDraw;
var size = 32;
function mapDraw() {
for (var i = 0; i < mapArray.length; i++) {
for (var j = 0; j < mapArray[i].length; j++) {
switch (mapArray[i][j]) {
case 0:
context.drawImage(room, posX, posY, size, size);
break;
case 1:
context.drawImage(redBox, posX, posY, size, size);
break;
default:
break;
}
posX += size;
}
posY += size;
posX = 0;
}
}
</script>
</body>
</html>
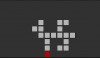
This is obviously a mockup and has no further functionality. I can script how to move the player around, but it would be nice to have something visual to represent it. No clue how others are achieving this in SugarCube. Any thoughts and ideas would be greatly appreciated.
Last edited: